Optimizing Your R programming assignments with Efficient SQL Query Writing
May 03, 2023
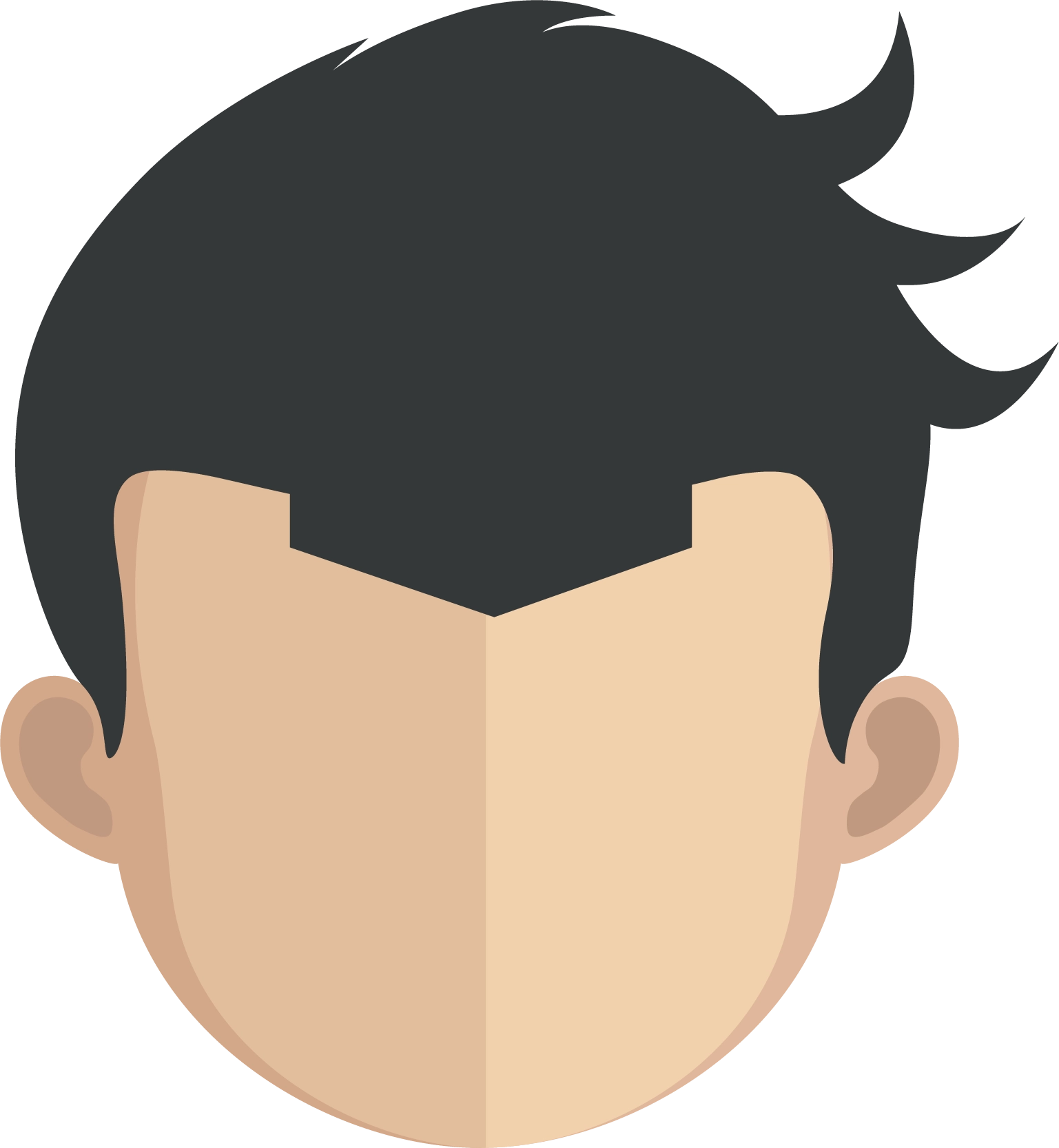
Michael Johan
United States of America
SQL Query
Michael Johan is a top-tier data analyst with Ph.D. in Data Science from Cornell University who has worked with SQL, R, and database management for more than 8 years. He holds expertise about writing complex SQL queries, optimizing, and making reports that are useful.
When working with data, it is common practice to combine information obtained from a variety of sources in order to gain new perspectives or make well-informed decisions. However, if you do not have the appropriate tools and methods, data manipulation can be a difficult process. Structured Query Language (SQL) is extremely helpful in situations like these. The Structured Query Language (SQL) is a type of programming language that enables users to efficiently manage and manipulate relational databases. On the other hand, R is a programming language that is primarily utilized for computational statistics and graphical representations. In this article, we will concentrate on the process of writing SQL queries in R programming in order to make the process of data manipulation more approachable and effective. Learning how to write SQL queries in R programming can help you easily perform complex data manipulations, regardless of whether you are just starting out as a programmer or have years of experience under your belt. In this lesson, you'll learn the fundamentals of writing a basic SQL query in R programming assignment, and then we'll move on to investigating more advanced SQL queries to help you make the most of your data.
Getting Started with SQL and R Programming
In order to better understand how to write SQL queries in R programming, let's first quickly go over what SQL and R programming are.
Communication with relational databases is accomplished through the use of SQL. Tables, which are made up of rows and columns, are used by relational databases to store the data. Data can be retrieved from, updated in, and removed from these tables with the help of SQL. The SQL commands SELECT, INSERT, UPDATE, and DELETE are among the most frequently used.
R programming, on the other hand, is a programming language that is utilized for statistical computing as well as graphics. Data analysis and statistical modeling are two common applications for the programming language R. R programming comes with a wide variety of packages that simplify the processes of data manipulation and analysis.
Installing Necessary Packages
Before we can start writing SQL queries in R programming, we need to install some packages. The following packages are necessary for writing SQL queries in R programming:
- DBI: This package provides a common interface to various database systems, including MySQL, PostgreSQL, and SQLite.
- RSQLite: This package provides an interface to SQLite databases.
- dplyr: This package provides tools for working with data frames, which can be useful for manipulating data before and after running SQL queries.
To install these packages, you can use the following commands:
install.packages("DBI")
install.packages("RSQLite")
install.packages("dplyr")
Connecting to a Database
To write SQL queries in R programming, we need to first establish a connection to a database. In this example, we will use an SQLite database.
Here's how to establish a connection to an SQLite database:
Here's how to establish a connection to an SQLite database:
library(DBI)
library(RSQLite)
# Establish a connection to the database
con <- dbConnect(RSQLite::SQLite(), "mydatabase.sqlite")
Replace "mydatabase.sqlite" with the path to your SQLite database file. If the database file does not exist, SQLite will create a new one.
Writing a Simple SQL Query
Now that we have established a connection to the database, we can write a simple SQL query.
In this example, we will retrieve all the records from a table called "employees":
In this example, we will retrieve all the records from a table called "employees":
library(dplyr)
# Retrieve all records from the employees table
employees <- dbGetQuery(con, "SELECT * FROM employees")
This SQL query retrieves all the records from the "employees" table and stores them in a data frame called "employees".
Advanced SQL Queries in R Programming
In the last section, we learned how to use R programming to write simple SQL queries. Now, let's look at some more advanced SQL queries that you can use to change and analyze your data.
The JOIN statement is a useful SQL command to know. You can use this command to combine data from two or more tables based on a column or key that they all share. The LEFT JOIN and RIGHT JOIN commands return all the rows from one table and only the rows that match from the other table. The INNER JOIN command returns only the rows that match between the two tables.
The GROUP BY statement is another powerful SQL command. It lets you group your data by one or more columns and then use aggregate functions like SUM, COUNT, and AVERAGE on each group. This is helpful for making summaries of your data and finding patterns in it.
In R programming, you can also use many other advanced SQL queries to change and analyze your data, in addition to these commands. You can get good at using SQL commands to get insights from your data if you practice and try different things.
Now that we know how to write a simple SQL query in R programming, let's explore some more advanced SQL queries.
Joining Tables
One of the most powerful features of SQL is the ability to join tables. Joining tables allows us to combine data from multiple tables into a single result set.
In this example, we will join two tables: "employees" and "departments":
In this example, we will join two tables: "employees" and "departments":
# Join the employees and departments tables
employees_departments <- dbGetQuery(con, "
SELECT *
FROM employees
JOIN departments
ON employees.department_id = departments.department_id
")
This SQL query joins the "employees" and "departments" tables on the "department_id" column and retrieves all the columns from both tables.
Filtering Data
The capability of SQL to filter data based on certain conditions is another useful feature of this programming language. In this particular illustration, we will apply a filter to the "employees" table so that we only retrieve records for employees who earn more than the given threshold.
# Filter the employees table to only retrieve records for employees with a salary greater than 50,000
employees_filtered <- dbGetQuery(con, "
SELECT *
FROM employees
WHERE salary > 50000
")
This SQL query uses the WHERE clause to filter the "employees" table and retrieve only records where the "salary" column is greater than 50,000.
Aggregating Data
SQL also allows us to aggregate data, which means we can calculate summary statistics for a group of records.
In this example, we will calculate the average salary for each department:
In this example, we will calculate the average salary for each department:
# Calculate the average salary for each department
average_salary <- dbGetQuery(con, "
SELECT department_id, AVG(salary) AS average_salary
FROM employees
GROUP BY department_id
")
This SQL query uses the GROUP BY clause to group the records by department_id, and then uses the AVG function to calculate the average salary for each group.
Best Practices for Writing SQL Queries in R Programming
SQL queries in R Programming can manipulate and analyze data, as we've seen. To write queries that are efficient, accurate, and easy to understand, follow some best practices.
First and foremost, your queries must be well-organized. To simplify the query, use proper indentation and line breaks. Use descriptive aliases for tables and columns instead of generic ones like "t1" or "col1." This will simplify your code and reduce errors.
When querying large datasets, use proper indexing and sorting. This can improve query performance and reduce result return time. It's also crucial to test and optimize queries to maximize efficiency.
Finally, SQL and R programming trends and best practices must be followed. This can keep you ahead of the curve and make your queries more effective. Following these best practices and staying current with SQL and R programming can make you a better data analyst.
Now that we have explored some advanced SQL queries in R programming, let's discuss some best practices for writing SQL queries in R Programming.
Parameterizing SQL Queries
When writing SQL queries, it is important to parameterize them to avoid SQL injection attacks. SQL injection attacks occur when an attacker inserts malicious SQL code into a query, which can cause the database to execute unintended commands. To parameterize a SQL query, you can use placeholders for the values that will be passed in.
Here's an example:
Here's an example:
# Parameterized SQL query
query <- "SELECT * FROM employees WHERE salary > ?"
employees_filtered <- dbGetQuery(con, query, params = list(50000))
In this example, the placeholder "?" is used to indicate where the value will be inserted. The actual value is passed in as a parameter using the "params" argument.
Using Indexes
Indexes can improve the performance of SQL queries by allowing the database to quickly locate the data that is needed. When writing SQL queries, it is important to use indexes where appropriate. Indexes can be created on columns that are frequently used in WHERE clauses, JOIN clauses, and ORDER BY clauses.
Here's an example of creating an index:
Here's an example of creating an index:
# Create an index on the salary column
dbExecute(con, "CREATE INDEX idx_salary ON employees (salary)")
In this example, an index is created on the "salary" column of the "employees" table.
Optimizing Queries
SQL queries can sometimes be slow, especially if they involve large tables or complex joins. When writing SQL queries, it is important to optimize them to improve performance. Here are some tips for optimizing queries:
- Use indexes where appropriate
- Avoid using SELECT * and only retrieve the columns that are needed
- Use WHERE clauses to filter data before performing joins
- Avoid using subqueries if possible
- Use the EXPLAIN command to analyze the query execution plan and identify bottlenecks
Conclusion
In this blog post, we talked about everything you need to know about writing a SQL query in R programming assignment. We started with the basics so that even people who had never done it before could follow along. We talked about what SQL and R programming are and why people often use them together. Then we learned how to connect to a database and write simple SQL queries. We showed how to use SQL functions like JOIN, WHERE, and GROUP BY to manipulate data and run complex queries.
Moreover, we also discussed some more advanced SQL queries, such as filtering data, aggregating data, and parameterizing queries. We gave real-world examples, tips, and tricks to make complex queries easier to understand. We also talked about the best ways to improve the performance of SQL queries in R programming, such as by indexing and optimizing queries.
By the end of this detailed guide, you should be able to write powerful SQL queries in R programming and handle data efficiently. If you know more about how SQL and R programming work together, you'll be able to make more complicated queries and show your professors how smart you are when you turn in your assignments.